mirror of
https://github.com/oqtane/oqtane.framework.git
synced 2025-05-19 11:04:23 +00:00
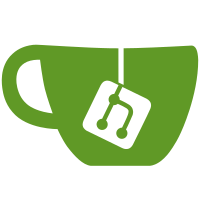
Centralized the code used to determine the available themes, panes, and containers. This isn't the most "ideal" way to handle this. However, it does improve the management of the code by centralizing the logic for theme selection. Future PR's development might improve this more.
101 lines
4.1 KiB
C#
101 lines
4.1 KiB
C#
using Oqtane.Models;
|
|
using System.Collections.Generic;
|
|
using System.Linq;
|
|
using System.Net.Http;
|
|
using System.Threading.Tasks;
|
|
using Microsoft.AspNetCore.Components;
|
|
using System.Reflection;
|
|
using System;
|
|
using Oqtane.Shared;
|
|
|
|
namespace Oqtane.Services
|
|
{
|
|
public class ThemeService : ServiceBase, IThemeService
|
|
{
|
|
private readonly HttpClient http;
|
|
private readonly string apiurl;
|
|
|
|
private List<Theme> themes;
|
|
|
|
public ThemeService(HttpClient http, IUriHelper urihelper)
|
|
{
|
|
this.http = http;
|
|
apiurl = CreateApiUrl(urihelper.GetAbsoluteUri(), "Theme");
|
|
}
|
|
//TODO: Implement Caching or otherwise on this, and other calls within this class
|
|
public async Task<List<Theme>> GetThemesAsync()
|
|
{
|
|
if (themes == null)
|
|
{
|
|
themes = await http.GetJsonAsync<List<Theme>>(apiurl);
|
|
|
|
// get list of loaded assemblies
|
|
Assembly[] assemblies = AppDomain.CurrentDomain.GetAssemblies();
|
|
|
|
foreach (Theme theme in themes)
|
|
{
|
|
if (theme.Dependencies != "")
|
|
{
|
|
foreach (string dependency in theme.Dependencies.Split(new char[] { ';' }, StringSplitOptions.RemoveEmptyEntries))
|
|
{
|
|
string assemblyname = dependency.Replace(".dll", "");
|
|
if (assemblies.Where(item => item.FullName.StartsWith(assemblyname + ",")).FirstOrDefault() == null)
|
|
{
|
|
// download assembly from server and load
|
|
var bytes = await http.GetByteArrayAsync("_framework/_bin/" + assemblyname + ".dll");
|
|
Assembly.Load(bytes);
|
|
}
|
|
}
|
|
}
|
|
if (assemblies.Where(item => item.FullName.StartsWith(theme.AssemblyName + ",")).FirstOrDefault() == null)
|
|
{
|
|
// download assembly from server and load
|
|
var bytes = await http.GetByteArrayAsync("_framework/_bin/" + theme.AssemblyName + ".dll");
|
|
Assembly.Load(bytes);
|
|
}
|
|
}
|
|
}
|
|
return themes.OrderBy(item => item.Name).ToList();
|
|
}
|
|
|
|
public Dictionary<string, string> CalculateSelectableThemes(List<Theme> themes)
|
|
{
|
|
var selectableThemes = new Dictionary<string, string>();
|
|
foreach (Theme theme in themes)
|
|
{
|
|
foreach (string themecontrol in theme.ThemeControls.Split(new char[] { ';' }, StringSplitOptions.RemoveEmptyEntries))
|
|
{
|
|
selectableThemes.Add(themecontrol, theme.Name + " - " + Utilities.GetTypeNameClass(themecontrol));
|
|
}
|
|
}
|
|
return selectableThemes;
|
|
}
|
|
|
|
public Dictionary<string, string> CalculateSelectablePaneLayouts(List<Theme> themes)
|
|
{
|
|
var selectablePaneLayouts = new Dictionary<string, string>();
|
|
foreach (Theme theme in themes)
|
|
{
|
|
foreach (string panelayout in theme.PaneLayouts.Split(new char[] { ';' }, StringSplitOptions.RemoveEmptyEntries))
|
|
{
|
|
selectablePaneLayouts.Add(panelayout, theme.Name + " - " + @Utilities.GetTypeNameClass(panelayout));
|
|
}
|
|
}
|
|
return selectablePaneLayouts;
|
|
}
|
|
|
|
public Dictionary<string, string> CalculateSelectableContainers(List<Theme> themes)
|
|
{
|
|
var selectableContainers = new Dictionary<string, string>();
|
|
foreach (Theme theme in themes)
|
|
{
|
|
foreach (string container in theme.ContainerControls.Split(new char[] { ';' }, StringSplitOptions.RemoveEmptyEntries))
|
|
{
|
|
selectableContainers.Add(container, theme.Name + " - " + @Utilities.GetTypeNameClass(container));
|
|
}
|
|
}
|
|
return selectableContainers;
|
|
}
|
|
}
|
|
}
|