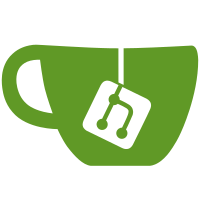
Fixes issue of unable to select information displayed from input and textarea changing the select property from disabled to readonly
213 lines
7.4 KiB
Plaintext
213 lines
7.4 KiB
Plaintext
@namespace Oqtane.Modules.Admin.Logs
|
|
@using System.Globalization
|
|
@inherits ModuleBase
|
|
@inject NavigationManager NavigationManager
|
|
@inject ILogService LogService
|
|
@inject IPageService PageService
|
|
@inject IPageModuleService PageModuleService
|
|
@inject IUserService UserService
|
|
|
|
<table class="table table-borderless">
|
|
<tr>
|
|
<td>
|
|
<Label For="dateTime" HelpText="The date and time of this log">Date/Time: </Label>
|
|
</td>
|
|
<td>
|
|
<input id="dateTime" class="form-control" @bind="@_logDate" readonly />
|
|
</td>
|
|
</tr>
|
|
<tr>
|
|
<td>
|
|
<Label For="level" HelpText="The level of this log">Level: </Label>
|
|
</td>
|
|
<td>
|
|
<input id="level" class="form-control" @bind="@_level" readonly />
|
|
</td>
|
|
</tr>
|
|
<tr>
|
|
<td>
|
|
<Label For="feature" HelpText="The feature that was affected">Feature: </Label>
|
|
</td>
|
|
<td>
|
|
<input id="feature" class="form-control" @bind="@_feature" readonly />
|
|
</td>
|
|
</tr>
|
|
<tr>
|
|
<td>
|
|
<Label For="function" HelpText="The function that was performed">Function: </Label>
|
|
</td>
|
|
<td>
|
|
<input id="function" class="form-control" @bind="@_function" readonly />
|
|
</td>
|
|
</tr>
|
|
<tr>
|
|
<td>
|
|
<Label For="category" HelpText="The categories that were affected">Category: </Label>
|
|
</td>
|
|
<td>
|
|
<input id="category" class="form-control" @bind="@_category" readonly />
|
|
</td>
|
|
</tr>
|
|
@if (_pageName != string.Empty)
|
|
{
|
|
<tr>
|
|
<td>
|
|
<Label For="page" HelpText="The page that was affected">Page: </Label>
|
|
</td>
|
|
<td>
|
|
<input id="page" class="form-control" @bind="@_pageName" readonly />
|
|
</td>
|
|
</tr>
|
|
}
|
|
@if (_moduleTitle != string.Empty)
|
|
{
|
|
<tr>
|
|
<td>
|
|
<Label For="module" HelpText="The module that was affected">Module: </Label>
|
|
</td>
|
|
<td>
|
|
<input id="module" class="form-control" @bind="@_moduleTitle" readonly />
|
|
</td>
|
|
</tr>
|
|
}
|
|
@if (_username != string.Empty)
|
|
{
|
|
<tr>
|
|
<td>
|
|
<Label For="user" HelpText="The user that caused this log">User: </Label>
|
|
</td>
|
|
<td>
|
|
<input id="user" class="form-control" @bind="@_username" readonly />
|
|
</td>
|
|
</tr>
|
|
}
|
|
<tr>
|
|
<td>
|
|
<Label For="url" HelpText="The url the log comes from">Url: </Label>
|
|
</td>
|
|
<td>
|
|
<input id="url" class="form-control" @bind="@_url" readonly />
|
|
</td>
|
|
</tr>
|
|
<tr>
|
|
<td>
|
|
<Label For="template" HelpText="What the log is about">Template: </Label>
|
|
</td>
|
|
<td>
|
|
<input id="template" class="form-control" @bind="@_template" readonly />
|
|
</td>
|
|
</tr>
|
|
<tr>
|
|
<td>
|
|
<Label For="message" HelpText="The message that the system generated"class="control-label">Message: </Label>
|
|
</td>
|
|
<td>
|
|
<textarea id="message" class="form-control" @bind="@_message" rows="5" readonly></textarea>
|
|
</td>
|
|
</tr>
|
|
@if (!string.IsNullOrEmpty(_exception))
|
|
{
|
|
<tr>
|
|
<td>
|
|
<Label For="exception" HelpText="The exceptions generated by the system">Exception: </Label>
|
|
</td>
|
|
<td>
|
|
<textarea id="exception" class="form-control" @bind="@_exception" rows="5" readonly></textarea>
|
|
</td>
|
|
</tr>
|
|
}
|
|
<tr>
|
|
<td>
|
|
<Label For="properties" HelpText="The properties that were affected">Properties: </Label>
|
|
</td>
|
|
<td>
|
|
<textarea id="properties" class="form-control" @bind="@_properties" rows="5" readonly></textarea>
|
|
</td>
|
|
</tr>
|
|
<tr>
|
|
<td>
|
|
<Label For="server" HelpText="The server that was affected">Server: </Label>
|
|
</td>
|
|
<td>
|
|
<input id="server" class="form-control" @bind="@_server" readonly />
|
|
</td>
|
|
</tr>
|
|
</table>
|
|
<NavLink class="btn btn-secondary" href="@NavigateUrl()">Cancel</NavLink>
|
|
|
|
@code {
|
|
private int _logId;
|
|
private string _logDate = string.Empty;
|
|
private string _level = string.Empty;
|
|
private string _feature = string.Empty;
|
|
private string _function = string.Empty;
|
|
private string _category = string.Empty;
|
|
private string _pageName = string.Empty;
|
|
private string _moduleTitle = string.Empty;
|
|
private string _username = string.Empty;
|
|
private string _url = string.Empty;
|
|
private string _template = string.Empty;
|
|
private string _message = string.Empty;
|
|
private string _exception = string.Empty;
|
|
private string _properties = string.Empty;
|
|
private string _server = string.Empty;
|
|
|
|
public override SecurityAccessLevel SecurityAccessLevel => SecurityAccessLevel.Host;
|
|
|
|
protected override async Task OnInitializedAsync()
|
|
{
|
|
try
|
|
{
|
|
_logId = Int32.Parse(PageState.QueryString["id"]);
|
|
var log = await LogService.GetLogAsync(_logId);
|
|
if (log != null)
|
|
{
|
|
_logDate = log.LogDate.ToString(CultureInfo.CurrentCulture);
|
|
_level = log.Level;
|
|
_feature = log.Feature;
|
|
_function = log.Function;
|
|
_category = log.Category;
|
|
|
|
if (log.PageId != null)
|
|
{
|
|
var page = await PageService.GetPageAsync(log.PageId.Value);
|
|
if (page != null)
|
|
{
|
|
_pageName = page.Name;
|
|
}
|
|
}
|
|
|
|
if (log.PageId != null && log.ModuleId != null)
|
|
{
|
|
var pagemodule = await PageModuleService.GetPageModuleAsync(log.PageId.Value, log.ModuleId.Value);
|
|
if (pagemodule != null)
|
|
{
|
|
_moduleTitle = pagemodule.Title;
|
|
}
|
|
}
|
|
|
|
if (log.UserId != null)
|
|
{
|
|
var user = await UserService.GetUserAsync(log.UserId.Value, PageState.Site.SiteId);
|
|
if (user != null)
|
|
{
|
|
_username = user.Username;
|
|
}
|
|
}
|
|
|
|
_url = log.Url;
|
|
_template = log.MessageTemplate;
|
|
_message = log.Message;
|
|
_exception = log.Exception;
|
|
_properties = log.Properties;
|
|
_server = log.Server;
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
await logger.LogError(ex, "Error Loading Log {LogId} {Error}", _logId, ex.Message);
|
|
AddModuleMessage("Error Loading Log", MessageType.Error);
|
|
}
|
|
}
|
|
}
|