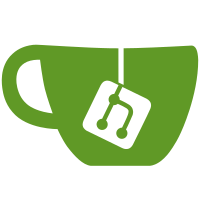
Add the option "Both" to display the toolbar at the top and bottom of the pager. Nice if the Pager is displaying large sets of data.
315 lines
10 KiB
Plaintext
315 lines
10 KiB
Plaintext
@namespace Oqtane.Modules.Controls
|
|
@inherits ModuleControlBase
|
|
@typeparam TableItem
|
|
|
|
@if (ItemList != null)
|
|
{
|
|
@if ((Toolbar == "Top" || Toolbar == "Both") && _pages > 0 && Items.Count() > _maxItems)
|
|
{
|
|
<ul class="pagination justify-content-center my-2">
|
|
<li class="page-item@((_page > 1) ? "" : " disabled")">
|
|
<a class="page-link" @onclick=@(async () => UpdateList(1))><span class="oi oi-media-step-backward" title="start" aria-hidden="true"></span></a>
|
|
</li>
|
|
@if (_pages > _displayPages && _displayPages > 1)
|
|
{
|
|
<li class="page-item@((_page > _displayPages) ? "" : " disabled")">
|
|
<a class="page-link" @onclick=@(async () => SkipPages("back"))><span class="oi oi-media-skip-backward" title="skip back" aria-hidden="true"></span></a>
|
|
</li>
|
|
}
|
|
<li class="page-item@((_page > 1) ? "" : " disabled")">
|
|
<a class="page-link" @onclick=@(async () => NavigateToPage("previous"))><span class="oi oi-chevron-left" title="previous" aria-hidden="true"></span></a>
|
|
</li>
|
|
@for (int i = _startPage; i <= _endPage; i++)
|
|
{
|
|
var pager = i;
|
|
if (pager == _page)
|
|
{
|
|
<li class="page-item active">
|
|
<a class="page-link" @onclick=@(async () => UpdateList(pager))>@pager</a>
|
|
</li>
|
|
}
|
|
else
|
|
{
|
|
<li class="page-item">
|
|
<a class="page-link" @onclick=@(async () => UpdateList(pager))>@pager</a>
|
|
</li>
|
|
}
|
|
}
|
|
<li class="page-item@((_page < _pages) ? "" : " disabled")">
|
|
<a class="page-link" @onclick=@(async () => NavigateToPage("next"))><span class="oi oi-chevron-right" title="next" aria-hidden="true"></span></a>
|
|
</li>
|
|
@if (_pages > _displayPages && _displayPages > 1)
|
|
{
|
|
<li class="page-item@((_endPage < _pages) ? "" : " disabled")">
|
|
<a class="page-link" @onclick=@(async () => SkipPages("forward"))><span class="oi oi-media-skip-forward" title="skip forward" aria-hidden="true"></span></a>
|
|
</li>
|
|
}
|
|
<li class="page-item@((_page < _pages) ? "" : " disabled")">
|
|
<a class="page-link" @onclick=@(async () => UpdateList(_pages))><span class="oi oi-media-step-forward" title="end" aria-hidden="true"></span></a>
|
|
</li>
|
|
<li class="page-item disabled">
|
|
<a class="page-link" style="white-space: nowrap;">Page @_page of @_pages</a>
|
|
</li>
|
|
</ul>
|
|
}
|
|
@if (Format == "Table" && Row != null)
|
|
{
|
|
<table class="@Class">
|
|
<thead>
|
|
<tr>@Header</tr>
|
|
</thead>
|
|
<tbody>
|
|
@foreach (var item in ItemList)
|
|
{
|
|
<tr>@Row(item)</tr>
|
|
@if (Detail != null)
|
|
{
|
|
<tr>@Detail(item)</tr>
|
|
}
|
|
}
|
|
</tbody>
|
|
</table>
|
|
}
|
|
@if (Format == "Grid" && Row != null)
|
|
{
|
|
int count = 0;
|
|
if (ItemList != null)
|
|
{
|
|
count = (int)Math.Ceiling(ItemList.Count() / (decimal)_columns) * _columns;
|
|
}
|
|
<div class="@Class">
|
|
@if (Header != null)
|
|
{
|
|
<div class="row"><div class="col">@Header</div></div>
|
|
}
|
|
@for (int row = 0; row < (count / _columns); row++)
|
|
{
|
|
<div class="row">
|
|
@for (int col = 0; col < _columns; col++)
|
|
{
|
|
int index = (row * _columns) + col;
|
|
if (index < ItemList.Count())
|
|
{
|
|
<div class="col">@Row(ItemList.ElementAt(index))</div>
|
|
}
|
|
else
|
|
{
|
|
<div class="col"> </div>
|
|
}
|
|
}
|
|
</div>
|
|
}
|
|
</div>
|
|
}
|
|
@if ((Toolbar == "Bottom" || Toolbar == "Both") && _pages > 0 && Items.Count() > _maxItems)
|
|
{
|
|
<ul class="pagination justify-content-center my-2">
|
|
<li class="page-item@((_page > 1) ? "" : " disabled")">
|
|
<a class="page-link" @onclick=@(async () => UpdateList(1))><span class="oi oi-media-step-backward" title="start" aria-hidden="true"></span></a>
|
|
</li>
|
|
@if (_pages > _displayPages)
|
|
{
|
|
<li class="page-item@((_page > _displayPages) ? "" : " disabled")">
|
|
<a class="page-link" @onclick=@(async () => SkipPages("back"))><span class="oi oi-media-skip-backward" title="skip back" aria-hidden="true"></span></a>
|
|
</li>
|
|
}
|
|
<li class="page-item@((_page > 1) ? "" : " disabled")">
|
|
<a class="page-link" @onclick=@(async () => NavigateToPage("previous"))><span class="oi oi-chevron-left" title="previous" aria-hidden="true"></span></a>
|
|
</li>
|
|
@for (int i = _startPage; i <= _endPage; i++)
|
|
{
|
|
var pager = i;
|
|
if (pager == _page)
|
|
{
|
|
<li class="page-item active">
|
|
<a class="page-link" @onclick=@(async () => UpdateList(pager))>@pager</a>
|
|
</li>
|
|
}
|
|
else
|
|
{
|
|
<li class="page-item">
|
|
<a class="page-link" @onclick=@(async () => UpdateList(pager))>@pager</a>
|
|
</li>
|
|
}
|
|
}
|
|
<li class="page-item@((_page < _pages) ? "" : " disabled")">
|
|
<a class="page-link" @onclick=@(async () => NavigateToPage("next"))><span class="oi oi-chevron-right" title="next" aria-hidden="true"></span></a>
|
|
</li>
|
|
@if (_pages > _displayPages)
|
|
{
|
|
<li class="page-item@((_endPage < _pages) ? "" : " disabled")">
|
|
<a class="page-link" @onclick=@(async () => SkipPages("forward"))><span class="oi oi-media-skip-forward" title="skip forward" aria-hidden="true"></span></a>
|
|
</li>
|
|
}
|
|
<li class="page-item@((_page < _pages) ? "" : " disabled")">
|
|
<a class="page-link" @onclick=@(async () => UpdateList(_pages))><span class="oi oi-media-step-forward" title="end" aria-hidden="true"></span></a>
|
|
</li>
|
|
<li class="page-item disabled">
|
|
<a class="page-link">Page @_page of @_pages</a>
|
|
</li>
|
|
</ul>
|
|
}
|
|
}
|
|
|
|
@code {
|
|
private int _pages = 0;
|
|
private int _page = 1;
|
|
private int _maxItems = 10;
|
|
private int _displayPages = 5;
|
|
private int _startPage = 0;
|
|
private int _endPage = 0;
|
|
private int _columns = 1;
|
|
|
|
[Parameter]
|
|
public string Format { get; set; } // Table or Grid
|
|
|
|
[Parameter]
|
|
public string Toolbar { get; set; } // Top, Bottom or Both
|
|
|
|
[Parameter]
|
|
public RenderFragment Header { get; set; } = null;
|
|
|
|
[Parameter]
|
|
public RenderFragment<TableItem> Row { get; set; } = null;
|
|
|
|
[Parameter]
|
|
public RenderFragment<TableItem> Detail { get; set; } = null; // only applicable to Table layouts
|
|
|
|
[Parameter]
|
|
public IEnumerable<TableItem> Items { get; set; } // the IEnumerable data source
|
|
|
|
[Parameter]
|
|
public string PageSize { get; set; } // number of items to display on a page
|
|
|
|
[Parameter]
|
|
public string Columns { get; set; } // only applicable to Grid layouts
|
|
|
|
[Parameter]
|
|
public string CurrentPage { get; set; } // optional property to set the initial page to display
|
|
|
|
[Parameter]
|
|
public string DisplayPages { get; set; } // maximum number of page numbers to display for user selection
|
|
|
|
[Parameter]
|
|
public string Class { get; set; }
|
|
|
|
private IEnumerable<TableItem> ItemList { get; set; }
|
|
|
|
protected override void OnParametersSet()
|
|
{
|
|
if (string.IsNullOrEmpty(Format))
|
|
{
|
|
Format = "Table";
|
|
}
|
|
|
|
if (string.IsNullOrEmpty(Toolbar))
|
|
{
|
|
Toolbar = "Top";
|
|
}
|
|
|
|
if (string.IsNullOrEmpty(Class))
|
|
{
|
|
if (Format == "Table")
|
|
{
|
|
Class = "table table-borderless";
|
|
}
|
|
else
|
|
{
|
|
Class = "container-fluid px-0";
|
|
}
|
|
}
|
|
|
|
if (!string.IsNullOrEmpty(PageSize))
|
|
{
|
|
_maxItems = int.Parse(PageSize);
|
|
}
|
|
|
|
if (!string.IsNullOrEmpty(Columns))
|
|
{
|
|
_columns = int.Parse(Columns);
|
|
}
|
|
|
|
if (!string.IsNullOrEmpty(DisplayPages))
|
|
{
|
|
_displayPages = int.Parse(DisplayPages);
|
|
}
|
|
|
|
if (!string.IsNullOrEmpty(CurrentPage))
|
|
{
|
|
_page = int.Parse(CurrentPage);
|
|
}
|
|
else
|
|
{
|
|
_page = 1;
|
|
}
|
|
|
|
_startPage = 0;
|
|
_endPage = 0;
|
|
|
|
if (Items != null)
|
|
{
|
|
_pages = (int)Math.Ceiling(Items.Count() / (decimal)_maxItems);
|
|
if (_page > _pages)
|
|
{
|
|
_page = _pages;
|
|
}
|
|
ItemList = Items.Skip((_page - 1) * _maxItems).Take(_maxItems);
|
|
SetPagerSize();
|
|
}
|
|
}
|
|
|
|
public void SetPagerSize()
|
|
{
|
|
_startPage = ((_page - 1) / _displayPages) * _displayPages + 1;
|
|
_endPage = _startPage + _displayPages - 1;
|
|
if (_endPage > _pages)
|
|
{
|
|
_endPage = _pages;
|
|
}
|
|
StateHasChanged();
|
|
}
|
|
|
|
public void UpdateList(int page)
|
|
{
|
|
ItemList = Items.Skip((page - 1) * _maxItems).Take(_maxItems);
|
|
_page = page;
|
|
SetPagerSize();
|
|
}
|
|
|
|
public void SkipPages(string direction)
|
|
{
|
|
switch (direction)
|
|
{
|
|
case "forward":
|
|
_page = _endPage + 1;
|
|
break;
|
|
case "back":
|
|
_page = _startPage - 1;
|
|
break;
|
|
}
|
|
|
|
SetPagerSize();
|
|
}
|
|
|
|
public void NavigateToPage(string direction)
|
|
{
|
|
switch (direction)
|
|
{
|
|
case "next":
|
|
if (_page < _pages)
|
|
{
|
|
_page += 1;
|
|
}
|
|
break;
|
|
case "previous":
|
|
if (_page > 1)
|
|
{
|
|
_page -= 1;
|
|
}
|
|
break;
|
|
}
|
|
|
|
UpdateList(_page);
|
|
}
|
|
}
|